Coding and Testing Tips -
This section contains development and testing tips that we use when working on Microsoft CRM projects. Hope you find them useful...
Microsoft .NET Framework version 2.0 versus version 1.1
Application Mode and Loader.aspx
Enabling the default Internet Explorer context menu
Accessing query string parameters
Referencing the Microsoft CRM assemblies or files
Authentication and coding with filtered views
Web.config file considerations
Server assembly impersonations
Authentication to test different users and roles
Enabling platform-level tracing
Enabling development errors
Microsoft .NET Framework Version 2.0
As documented in the SDK, Microsoft CRM 3.0 does support writing client-side code using Visual Studio .NET 2005 and the .NET Framework version 2.0 that accesses the CRMService and MetadataService Web services. However, Microsoft CRM 3.0 does not support callout or workflow assemblies written in Visual Studio .NET 2005 and the .NET Framework version 2.0. You should still continue to use Visual Studio .NET 2003 and the .NET Framework Version 1.1 when creating custom assemblies for callouts and workflow.
Please review the latest SDK for the most up-to-date information on the support for .NET Framework Version 2.0.
Application Mode and Loader.Aspx
Of course you've noticed that Microsoft CRM launches into a special Internet Explorer window that does not have the menu bar, address bar, toolbar, and so on. Microsoft CRM refers to this as the Application Mode, and it runs in this mode by default. Often, developers need these additional Internet Explorer features that application mode hides. You can launch Microsoft CRM in a standard Internet Explorer window by browsing to http://
Using the Loader.aspx page disables application mode for that single Web session. You can also permanently disable application mode for all users and all sessions by updating the Web.config file.
Disabling Application Mode
On the Microsoft CRM Web server, navigate to
Open the Web.config file in Notepad (or any text editor).
Look for the AppMode key, and change its value to Off.
Save the Web.config file.
Enabling the Default Internet Explorer Context Menu
In addition to running in Application Mode, Microsoft CRM modifies the standard Internet Explorer behavior by displaying its own context menu when you right-click in the application. Right-clicking a grid gives you options unique to Microsoft CRM, such as Open, Print, and Refresh List. However, right-clicking a form won't display a context menu like you would see on a normal Web page. When you're troubleshooting and debugging, you might find that you want to access the standard Internet Explorer context menu so that you can use features such as View Source, Properties, or Open In New Window. You can re-enable the Internet Explorer context menu by editing the Global.js file.
Re-Enabling the Internet Explorer Standard Context Menu
On the Microsoft CRM Web server, navigate to
Open the Global.js file in Notepad (or any text editor). Note: Do not double-click this file because it will attempt to execute the JavaScript file.
Right-click the file, and then click Edit.
Use your text editor's Find feature to locate the document.oncontextmenu() function.
Comment out the existing code in this function by adding /* and */ as shown in the following code. You can undo this change later by simply removing event.returnValue = true; line and the comment characters.
function document.oncontextmenu()
{
event.returnValue = true;
/*
var s = event.srcElement.tagName;
(!event.srcElement.disabled &&
(document.selection.createRange().text.length > 0
s == "TEXTAREA"
s == "INPUT" && event.srcElement.type == "text"));
*/
}
Save the file.
Open a page in Microsoft CRM and right-click it. You will see the familiar Internet Explorer context menu.
Caution Use this technique on development servers only. Do not modify the Global.js file in a production or staging environment; this unsupported change might cause unpredictable behavior. Microsoft CRM prevents use of the right-click context menu for the user's benefit and also to maintain a predictable navigation structure in the application interface.
Accessing Query String Parameters
When testing or debugging your code, you will frequently need the GUID of an entity record or want to see the query string parameters that Microsoft CRM passed to the window. You can access the URL of any page and view its GUID by pressing Ctrl+N on your keyboard. Internet Explorer opens a new window, and the address bar displays its query string parameters. You can also press the F11 key when viewing a record to toggle the display so that the URL address bar appears.
If you have re-enabled the Internet Explorer context menu, you can also get this information by right-clicking the page and selecting Properties. You can then copy the URL from the Properties dialog box.
Referencing the Microsoft CRM Assemblies or Files
You should never reference any Microsoft CRM assemblies other than Microsoft.Crm.Platform .Callout.Base.dll. You also should not import any of the JavaScript, style sheets, or behavior files into your project. Microsoft will not support this type of code reuse, and you will probably experience significant code problems when Microsoft CRM releases hotfixes, updates, or patches.
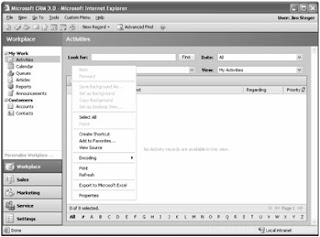
Caution Because you're not supposed to even reference any of the Microsoft CRM assemblies other than Microsoft.Crm.Platform.Callout.Base.dll, it should also be pretty obvious that you shouldn't attempt to modify any of the Microsoft CRM .dll files, either.
If you want to mimic any look or functionality of Microsoft CRM, you must re-create it yourself. The SDK provides a sample style sheet and a UI Style Guide, but if you want to review and understand the native script files, you can find most of them in <>\_common. If you want to review styles or code, copy these files to your own directory.
Authentication and Coding with Filtered Views
The SQL filtered views provide an excellent method for you to quickly and easily retrieve Microsoft CRM data by using standard SQL Server connections. However, because the filtered views rely on Integrated Windows authentication for data security, you must pay attention to how your code connects to the filtered views.
If you were to go under the hood of a filtered view in SQL and look at its script, you would see that it uses a custom function to enforce the security settings.
create function dbo.fn_FindUserGuid ()
returns uniqueidentifier
as
begin
declare @userguid uniqueidentifier
select @userguid = s.SystemUserId
from SystemUserBase s
where s.DomainName = SUSER_SNAME()
return @userguid
end
SUSER_SNAME() is a special function in SQL that returns the currently authenticated user, and the preceding function uses it to find the systemuserid identifier. If your code doesn't authenticate to SQL Server by using Windows authentication, your queries will never return any data, because all of the filtered views perform an inner join using systemuserid.
To connect to the database by using Windows authentication instead of SQL Server authentication, your connection string should include the following parameter: Integrated Security=SSPI. The full string would look something like this.
server=databaseserver;database=yourcustomdatabase;Integrated Security=SSPI
More Info Remember that you should never alter the Microsoft CRM databases by adding your own routines or stored procedures. Microsoft doesn't support this type of alteration, and it will probably result in the loss of your routines upon upgrades or hotfixes. Instead, you should create your own database to store your custom routines.
If you're calling your custom SQL routines from a Web page, you must configure additional settings to make sure that your Web page passes the integrated Windows credentials to SQL Server. First, the user should belong to the SQLAccessGroup, which will happen automatically when you add users to Microsoft CRM. Second, you must ensure that your Web application does not permit anonymous access by disabling anonymous access in IIS for your virtual directory or Web site. Finally, you must add or update the Web.config file in your custom web with the following nodes located in the
These keys ensure that IIS uses the Windows credential set when accessing a page in your virtual web.
As we explained earlier, another consequence of the integrated security features in filtered views is that you can't use filtered views in callouts or in workflow assemblies. The callout and workflow engine runs under the security context of the user, and, because you cannot impersonate to SQL Server from your connection string, you cannot retrieve data by using the filtered views. In these cases, we recommend that you use the QueryExpression class because the Web service does allow impersonation.
Web.Config File Considerations
If you create custom pages that work with Microsoft CRM, you should deploy them to a directory outside of the Microsoft CRM root and create a new Web site or a new virtual directory. The easiest way to leverage cross-site scripting (see Chapter 10 for more details) is to set up a virtual directory underneath the Microsoft CRM web. When you create a virtual directory, you will inherit the Web.config settings of the parent web, which in this case would be settings from the Microsoft CRM Web.config file.
If you add your custom web files to a virtual directory underneath the Microsoft CRM web, you might receive an error similar to this one when accessing your custom pages:
"File or assembly name Microsoft.Crm.Tools.ImportExportPublish, or one of its dependencies, was not found."
You have a couple of options for getting around this error. One is to register each of the assemblies that fail in the global assembly cache (GAC). The other is to remove these entries in your custom Web.config file. To remove the assemblies from your Web.config file, add the
Warning Make sure that you add this to your Web.config file. Do not alter these values in the Microsoft CRM Web.config file.
Server Assembly Impersonation
You might find that you need to use the credentials of the user making the request in your callouts or workflow assemblies. The SDK documents this numerous times, but, because it is such a useful technique, we want to mention here, too. This code snippet shows how to accomplish impersonation.
CrmService service = new CrmService();
service.Credentials = System.Net.CredentialCache.DefaultCredentials;
service.Url = "http://
// Get the current user ID.
WhoAmIRequest userRequest = new WhoAmIRequest();
WhoAmIResponse userResp = (WhoAmIResponse) service.Execute(userRequest);
service.CallerIdValue = new CallerId();
service.CallerIdValue.CallerGuid = userResp.UserId;
When you are using impersonation from a callout, the user context already contains the user ID for you. So, instead of making an additional request, you could simply use the following.
CrmService service = new CrmService();
service.Credentials = System.Net.CredentialCache.DefaultCredentials;
service.Url = "http://
service.CallerIdValue = new CallerId();
service.CallerIdValue.CallerGuid = userContext.UserId;
Enabling Development Errors in the Web.Config File
On the Microsoft CRM Web server, navigate to
Open the Web.config file in Notepad (or any text editor).
Look for the DevErrors key, and change its value to On.
Save the Web.config file.
Thanks to Mike Snyder and Jim Steger for writing down these tips in their book. You can refer to their book - Working with Microsoft Dynamics CRM 3.0 for more details. Thanks - D
No comments:
Post a Comment